Introduction
OpenAI’s New Function Calling feature has recently been added to their updated models, GPT-4 and GPT-3.5. This intriguing capability enables users to provide Python function descriptions to the models and then ask queries based on those descriptions. If the models determine that one of the submitted function descriptions is necessary to answer the query, they will provide the required parameters to execute the function as stated.
This feature provides a tool usage similar to LangChain but within the OpenAI API, making it more convenient and efficient. In this blog, we will go over the specifics of this feature, as well as the implementation process and various samples.
Understanding Function Calling in GPT
In GPT-4 and GPT-3.5, function calling entails describing functions to the models. The name of the function, a brief explanation of its purpose, and its parameters are all included in these descriptions.
The models examine the question and evaluate if it requires the execution of a function. If a function call is required, the models deliver a JSON object containing the function’s arguments. It should be noted that the chat completions API does not actually execute the function; instead, it simply returns the appropriate parameters in JSON format.
Function Calling Implementation
To demonstrate the function calling feature, we will use a function called “page builder,” which generates product web pages. This function takes a title and copies text as inputs and writes them to an HTML file. Initially, we define the function and provide instructions to GPT-4 or GPT-3.5 on how to use it.
first, we will start by installing the required dependencies:
!pip install -qU "openai==0.27.8"
We import openai
and authenticate with a free API key:
import openai
openai.api_key = "YOUR_OPEN_AI_KEY"
now let us define a function that will create a page for a product:
from IPython.display import display, HTML
def page_builder(title: str, copy_text: str):
"""Takes title and copy text to create a product page in simple HTML
"""
html = """
<!DOCTYPE html>
<html>
<head>
<title>awesome product</title>
<style>
body {
font-family: 'Open Sans', sans-serif;
}
.section {
background-color: #fff;
text-color: #000;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
margin: 0 auto;
width: 80%;
}
.title {
font-size: 2rem;
font-weight: 700;
color: #000;
}
.container {
padding: 2rem;
}
.container p {
font-size: 1.2rem;
color: #d5d5d5;
}
</style>
</head>
<body>
<section class="section">
<div class="container">
<h1 class="title">""" + title + """</h1>
<p>""" + copy_text + """</p>
</div>
</section>
</body>
</html>
"""
with open('index.html', 'w') as fp:
fp.write(html)
return display(HTML(filename='index.html'))
now let us try to create a sample page:
page_builder(
title="Test",
copy_text="A sample copy text."
)

This looks great as a starting point now let’s create a function that open ai can understand.
page_builder_func = {
"name": "page_builder",
"description": "Creates product web pages",
"parameters": {
"type": "object",
"properties": {
"title": {
"type": "string",
"description": "The name of the product"
},
"copy_text": {
"type": "string",
"description": "Marketing copy that describes and sells the product"
}
},
"required": ["title", "copy_text"]
}
}
Now let us break it down and understand better.
The name as evidence describes the name of the tool or function. A point to note is that it does not need to be the same as the name of the function we defined earlier. The description describes what this function does.
Now let us focus on parameters, which are essentially the parameters that the function will use when it is passed on. In our case it contains Title which further contains its type defining the type of parameter needed and its description and similarly for the copy text parameter
Then we have a required field that informs OpenAI that these parameters must be provided.
Now let us run this:
prompt = "Create a web page for a new shoe shining Product"
res = openai.ChatCompletion.create(
model='gpt-3.5-turbo-0613', # swap for gpt-4-0613 if you have access to it
messages=[{"role": "user", "content": prompt}],
functions=[page_builder_func]
)
res
Output:
<OpenAIObject chat.completion id=chatcmpl-7Vvunj8dP07XbRhckhz1fm8w1bIJX at 0x7faad77890d0> JSON: {
"id": "chatcmpl-7Vvunj8dP07XbRhckhz1fm8w1bIJX",
"object": "chat.completion",
"created": 1687845149,
"model": "gpt-3.5-turbo-0613",
"choices": [
{
"index": 0,
"message": {
"role": "assistant",
"content": null,
"function_call": {
"name": "page_builder",
"arguments": "{\n \"title\": \"GleamShine Shoe Polish\",\n \"copy_text\": \"GleamShine Shoe Polish is the ultimate solution to keeping your shoes looking stylish and new. Our advanced formula not only restores color and shine, but also provides long-lasting protection against dirt, water, and scuffs. With GleamShine, your shoes will always make a lasting impression. Get ready to step up your shoe game with GleamShine Shoe Polish.\"\n}"
}
},
"finish_reason": "function_call"
}
],
"usage": {
"prompt_tokens": 76,
"completion_tokens": 103,
"total_tokens": 179
}
}
Now how do we know that the response that GPT sent us is complete that is it wants us to run the function with the parameters? When we get a response from the model, we look at the finish_reason property.
If it is a function call, we will extract the function name and arguments. Because we only have one function, it simplifies the process in our situation. The page_builder function converts the arguments into a dictionary and passes them as keyword arguments. This stage runs the function and creates the product web page, which includes the title, copy text, and an optional image.
now let us do that and it for ourselves:
import json
name = res['choices'][0]['message']['function_call']['name']
args = json.loads(res['choices'][0]['message']['function_call']['arguments'])
name, args
Now we have our function arguments in the args dictionary let us execute it:
page_builder(**args)
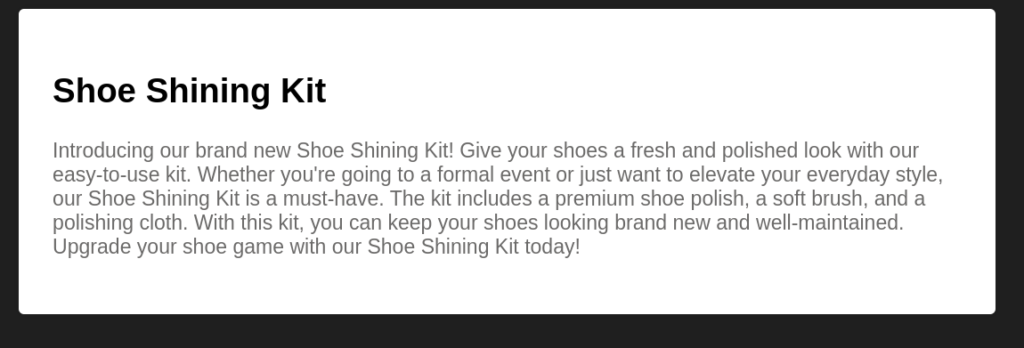
So it generated output for us now. Similarly, we can create different functions which take different parameters.
Conclusion
In conclusion, we can say that OpenAI’s new function calling feature will definitely help us further create powerful functions and applications.